Last exercise "
Convert between Uri and file path, and load Bitmap from" load picture with intent of "Intent.ACTION_PICK". It's modify to do the similary with "Intent.ACTION_GET_CONTENT".
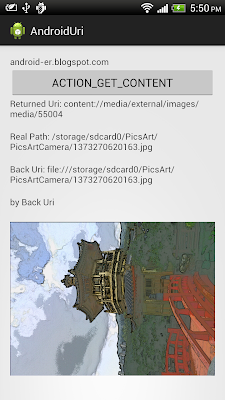
package com.example.androiduri;
import java.io.File;
import java.io.FileNotFoundException;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.content.CursorLoader;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.app.Activity;
import android.content.Intent;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
public class MainActivity extends Activity {
Button btnSelFile;
TextView text1, text2, text3, note;
ImageView image;
Uri orgUri, uriFromPath;
String convertedPath;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btnSelFile = (Button)findViewById(R.id.selfile);
text1 = (TextView)findViewById(R.id.text1);
text2 = (TextView)findViewById(R.id.text2);
text3 = (TextView)findViewById(R.id.text3);
note = (TextView)findViewById(R.id.note);
image = (ImageView)findViewById(R.id.image);
btnSelFile.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View arg0) {
Intent intent = new Intent(Intent.ACTION_GET_CONTENT);
Uri data = Uri.fromFile(Environment.getExternalStorageDirectory());
String type = "image/*";
intent.setDataAndType(data, type);
startActivityForResult(intent, 0);
}});
text1.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
image.setImageBitmap(null);
note.setText("by Returned Uri");
try {
Bitmap bm = BitmapFactory.decodeStream(
getContentResolver().openInputStream(orgUri));
image.setImageBitmap(bm);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}});
text2.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
image.setImageBitmap(null);
note.setText("by Real Path");
Bitmap bm = BitmapFactory.decodeFile(convertedPath);
image.setImageBitmap(bm);
}});
text3.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
image.setImageBitmap(null);
note.setText("by Back Uri");
try {
Bitmap bm = BitmapFactory.decodeStream(
getContentResolver().openInputStream(uriFromPath));
image.setImageBitmap(bm);
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == RESULT_OK){
image.setImageBitmap(null);
note.setText("");
//Uri return from external activity
orgUri = data.getData();
if(orgUri != null){
text1.setText("Returned Uri: " + orgUri.toString() + "\n");
//path converted from Uri
convertedPath = getRealPathFromURI(orgUri);
if(convertedPath != null){
text2.setText("Real Path: " + convertedPath + "\n");
//Uri convert back again from path
uriFromPath = Uri.fromFile(new File(convertedPath));
text3.setText("Back Uri: " + uriFromPath.toString() + "\n");
}else{
text2.setText("Real Path: " + "null");
text3.setText("");
}
}else{
text1.setText("Returned Uri: " + "null");
text2.setText("");
text3.setText("");
}
}
}
public String getRealPathFromURI(Uri contentUri) {
String[] proj = { MediaStore.Images.Media.DATA };
String result = null;
CursorLoader cursorLoader = new CursorLoader(
this,
contentUri, proj, null, null, null);
Cursor cursor = cursorLoader.loadInBackground();
if(cursor != null){
int column_index =
cursor.getColumnIndexOrThrow(MediaStore.Images.Media.DATA);
cursor.moveToFirst();
result = cursor.getString(column_index);
}
return result;
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical"
tools:context=".MainActivity" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="android-er.blogspot.com" />
<Button
android:id="@+id/selfile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ACTION_GET_CONTENT" />
<TextView
android:id="@+id/text1"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/text2"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/text3"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/note"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
Download the files.