Last example demonstrate a "
Simple example of Button and OnClickListener", with individual OnClickListener for each View. Alternatively, we can have a common OnClickListener for more than one View, then determine event source in event listener, and perform the correspond operation accordingly.
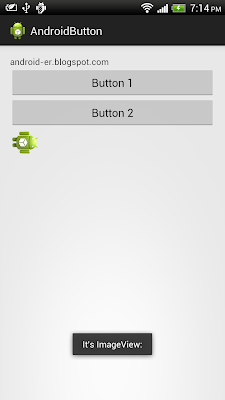
package com.example.androidbutton;
import android.os.Bundle;
import android.app.Activity;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity implements OnClickListener{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
LinearLayout myBackGround = (LinearLayout)findViewById(R.id.mybackground);
myBackGround.setOnClickListener(this);
TextView myWeb = (TextView)findViewById(R.id.myweb);
myWeb.setOnClickListener(this);
Button button1 = (Button)findViewById(R.id.button1);
button1.setOnClickListener(this);
Button button2 = (Button)findViewById(R.id.button2);
button2.setOnClickListener(this);
ImageView myImage =(ImageView)findViewById(R.id.myimage);
myImage.setOnClickListener(this);
}
@Override
public void onClick(View v) {
if(v.getClass() == TextView.class){
Toast.makeText(getApplicationContext(),
"It's TextView:\n" + ((TextView)v).getText(),
Toast.LENGTH_SHORT).show();
}else if(v.getClass() == Button.class){
Toast.makeText(getApplicationContext(),
"It's Button:\n" + ((Button)v).getText(),
Toast.LENGTH_SHORT).show();
}else if(v.getClass() == ImageView.class){
Toast.makeText(getApplicationContext(),
"It's ImageView:",
Toast.LENGTH_SHORT).show();
//setRotation require API Level 11
float rot = ((ImageView)v).getRotation() + 90;
((ImageView)v).setRotation(rot);
}else{
Toast.makeText(getApplicationContext(),
"Background clicked",
Toast.LENGTH_SHORT).show();
}
}
}
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical"
tools:context=".MainActivity"
android:id="@+id/mybackground" >
<TextView
android:id="@+id/myweb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="android-er.blogspot.com" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button 1"/>
<Button
android:id="@+id/button2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Button 2"/>
<ImageView
android:id="@+id/myimage"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher"/>
</LinearLayout>